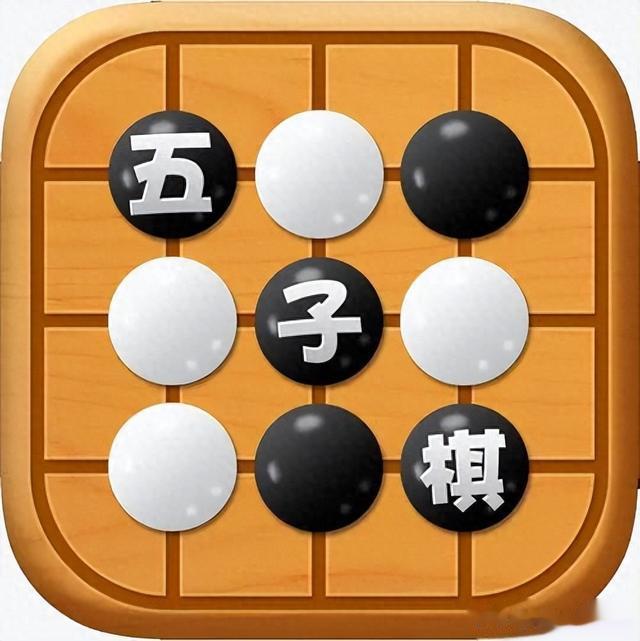
实现一个五子棋(Gomoku)游戏使用Java Swing涉及多个步骤。以下是一个简化的五子棋游戏实现,包括基本的游戏逻辑和图形用户界面(GUI)。
游戏逻辑:一个15x15的棋盘(为了简化,通常的棋盘是19x19,但这里我们使用15x15)。两种颜色的棋子:黑色和白色。交替下棋。检查五子连珠。GUI:使用JPanel来显示棋盘。使用JButton来表示棋盘上的每一个交叉点。以下是一个简化的实现:
import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public GomokuGame extends JFrame { private static final int SIZE = 15; private static final int CELL_SIZE = 30; private JButton[][] buttons = new JButton[SIZE][SIZE]; private int currentPlayer = 0; // 0 for black, 1 for white public GomokuGame() { setTitle("Gomoku Game"); setSize(SIZE * CELL_SIZE, SIZE * CELL_SIZE); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JPanel boardPanel = new JPanel(new GridLayout(SIZE, SIZE)); for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { JButton btn = new JButton(); btn.setPreferredSize(new Dimension(CELL_SIZE, CELL_SIZE)); btn.addActionListener(new ButtonListener(i, j)); boardPanel.add(btn); buttons[i][j] = btn; } } add(boardPanel, BorderLayout.CENTER); setVisible(true); } private ButtonListener implements ActionListener { private int row, col; public ButtonListener(int row, int col) { this.row = row; this.col = col; } @Override public void actionPerformed(ActionEvent e) { JButton btn = (JButton) e.getSource(); if (currentPlayer == 0) { btn.setBackground(Color.BLACK); } else { btn.setBackground(Color.WHITE); } btn.setEnabled(false); if (checkWin(row, col)) { JOptionPane.showMessageDialog(null, "Player " + (currentPlayer + 1) + " wins!"); resetBoard(); } currentPlayer = (currentPlayer + 1) % 2; } } private boolean checkWin(int row, int col) { // Simplified check for horizontal, vertical, and one diagonal int player = currentPlayer == 0 ? 1 : -1; // Horizontal int count = 1; for (int i = 1; i < 5; i++) { if ((row + i < SIZE && buttons[row + i][col].getBackground().equals(buttons[row][col].getBackground()))) { count++; } else { break; } } for (int i = 1; i < 5; i++) { if ((row - i >= 0 && buttons[row - i][col].getBackground().equals(buttons[row][col].getBackground()))) { count++; } else { break; } } if (count >= 5) return true; // Vertical count = 1; for (int i = 1; i < 5; i++) { if ((col + i < SIZE && buttons[row][col + i].getBackground().equals(buttons[row][col].getBackground()))) { count++; } else { break; } } for (int i = 1; i < 5; i++) { if ((col - i >= 0 && buttons[row][col - i].getBackground().equals(buttons[row][col].getBackground()))) { count++; } else { break; } } if (count >= 5) return true; // Diagonal \ count = 1; for (int i = 1; i < 5; i++) { if ((row + i < SIZE && col + i < SIZE && buttons[row + i][col + i].getBackground().equals(buttons[row][col].getBackground()))) { count++; } else { break; } } for (int i = 1; i < 5; i++) { if ((row - i >= 0 && col - i >= 0 && buttons[row - i][col - i].getBackground().equals(buttons[row][col].getBackground()))) { count++; } else { break; } } if (count >= 5) return true; return false; } private void resetBoard() { for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { buttons[i][j].setBackground(Color.YELLOW); buttons[i][j].setEnabled(true); } } currentPlayer = 0; } public static void main(String[] args) { SwingUtilities.invokeLater(() -> new GomokuGame()); } }这只是一个非常简化的实现,仅用于演示基本的五子棋游戏逻辑和GUI。
在真实的五子棋游戏中,通常会有更多的功能,如悔棋、重新开始游戏、显示当前的玩家、优化UI等。